Import Infrastructure Objects via API
Import Infrastructure Objects from your IaaS account(s)
Infrastructure Catalog allows you to import Infrastructure Objects across your various IaaS accounts. Once imported, you can see details about your objects (source account, region, storage size, etc), filter ("how many databases do I have cumulatively across all my accounts?"), and define relationships between Infrastructure Objects to Services, Systems, and other Infrastructure Objects.
Create Infrastructure Objects via GraphQL API
Infrastructure Objects can be imported to OpsLevel from your IaaS account(s) via the GraphQL API using the mutation below:
mutation infrastructureResourceCreate(
$ownerId: ID,
$data: JSON,
$schema: InfrastructureResourceSchemaInput,
$providerData: InfrastructureResourceProviderDataInput,
$providerResourceType: String,
) {
infrastructureResourceCreate(
input: {
ownerId: $ownerId,
data: $data,
schema: $schema,
providerData: $providerData,
providerResourceType: $providerResourceType,
}
) {
infrastructureResource {
id
name
data
type
providerData {
accountName
externalUrl
providerName
}
providerResourceType
owner {
...on Team {
id
}
}
}
warnings {
message
}
errors {
message
path
}
}
}
We support a wide range of commonly used Infrastructure Objects, namely:
- Cache
- Compute
- Database
- Networking
- Queues / Message Brokers
- Serverless Function
- Storage
In order to determine the types of objects we support and the fields you can push for those types, you will need to use the query below:
query infrastructureResourceSchemas {
account {
infrastructureResourceSchemas {
nodes {
type
schema
}
}
}
}
If there is an Object not listed that you would be interested in importing, please reach out to our customer support team.
Example Query Variables
You can use the example query variables below with the infrastructureResourceCreate
mutation to create some examples. Not all of the fields are included, refer to the schema for available fields.
Compute
{
"data": {
"name": "gke-autoscale-prod-1-example",
"instance_id": "6412763931310739112",
"external_id": "https://www.googleapis.com/compute/v1/projects/genial-tangent-1234/zones/us-central1-c/instances/gke-autoscale-prod-1-example",
"image_id": "debian-11-bullseye-v20230615",
"zone": "us-central1-c",
"instance_type": "f1-micro",
"platform_details": "Debian GNU/Linux",
"launch_time": "2023-06-08T13:26:35.000000",
"public_ip_address": "--"
},
"schema": {"type": "Compute"},
"providerData": {
"accountName": "5604422034290",
"externalUrl": "https://www.googleapis.com/compute/v1/projects/genial-tangent-1234",
"providerName": "GCP"
},
"providerResourceType": "Compute Engine"
}
Database
{
"data": {
"name": "books-db-example",
"external_id": "https://sqladmin.googleapis.com/sql/v1beta4/projects/genial-tangent-1234/instances/books-db-example",
"zone": "us-central1",
"engine": "mySQL",
"engine_version": "8.0",
"endpoint": "genial-tangent-1234:us-central1:books-db-example",
"multi_zone_availability_enabled": true,
"publicly_accessible": false,
"port": 3306,
"zone": "us-central1",
"instance_type": "db-n1-standard-1",
"storage_size": {
"value": 200,
"unit": "GB"
},
"storage_encrypted": true,
"maintenance_window": "Monday",
"creation_date": "2023-06-07T22:01:21.417000Z"
},
"schema": {"type": "Database"},
"providerData": {
"accountName": "5604422034290",
"externalUrl": "https://console.cloud.google.com/home/dashboard?project=genial-tangent-1234",
"providerName": "GCP"
},
"providerResourceType": "SQL"
}
VPC
{
"data": {
"name": "gcp-vpc-5-example",
"external_id": "https://www.googleapis.com/compute/v1/projects/genial-tangent-1234/global/networks/gcp-vpc-5-example",
"zone": "global",
"subnets": [],
"is_default": true,
"internet_gateway": "--",
"dns_hostnames_enabled":false,
"ipv4_cidr": "--",
"nat_gateway": "--",
"dns_support_enabled":false
},
"schema": {"type": "Network"},
"providerData": {
"accountName": "5604422034290",
"externalUrl": "https://www.googleapis.com/compute/v1/projects/genial-tangent-1234",
"providerName": "GCP"
},
"providerResourceType": "VPC network"
}
Accessing Infrastructure objects
You can access your imported infrastructure objects by selecting Infrastructure in Catalog from the side navigation.
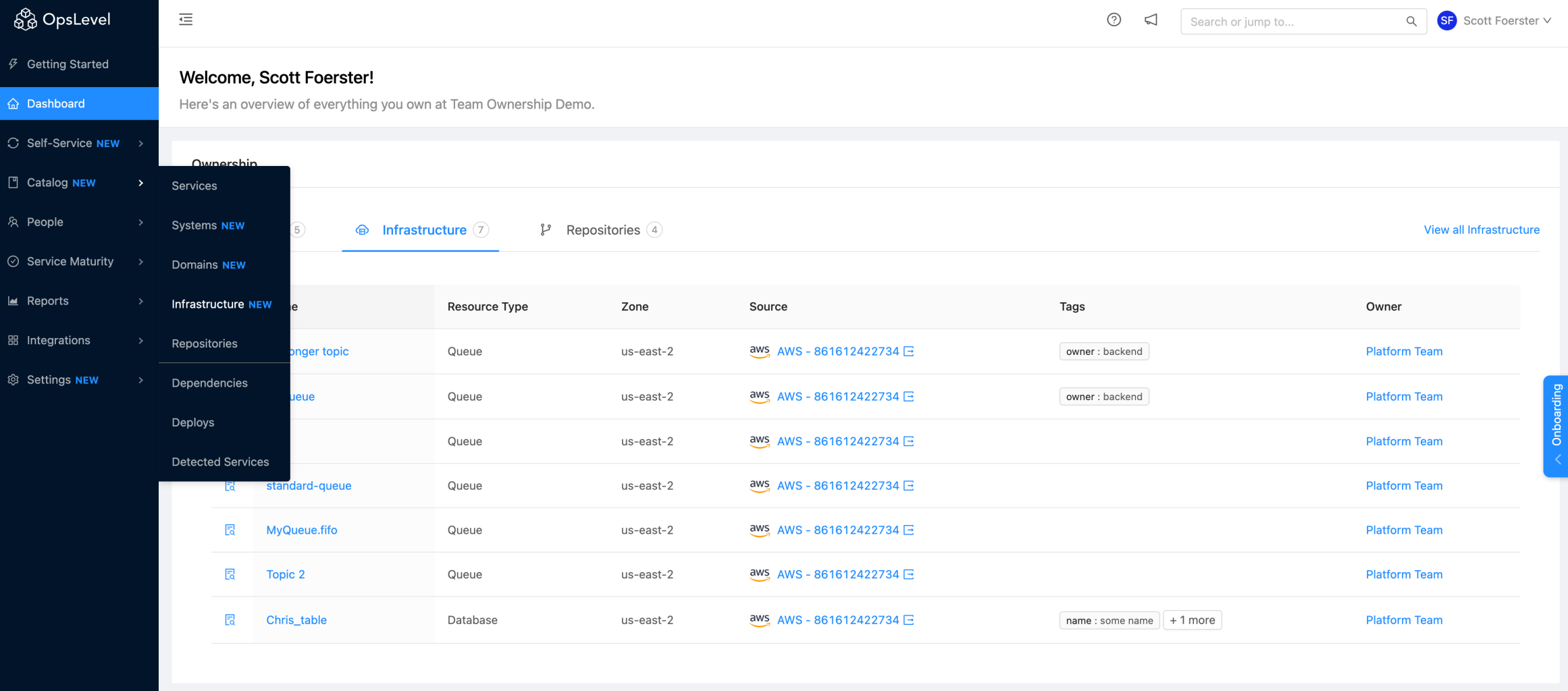
Infrastructure menu item
Once in the Infrastructure list page you will see a table listing all of the infrastructure objects you have imported. At a glance you will be able to see the following data around your objects:
- Name
- Type
- Provider Resource Type
- Zone
- Source
- Tags
- Owner
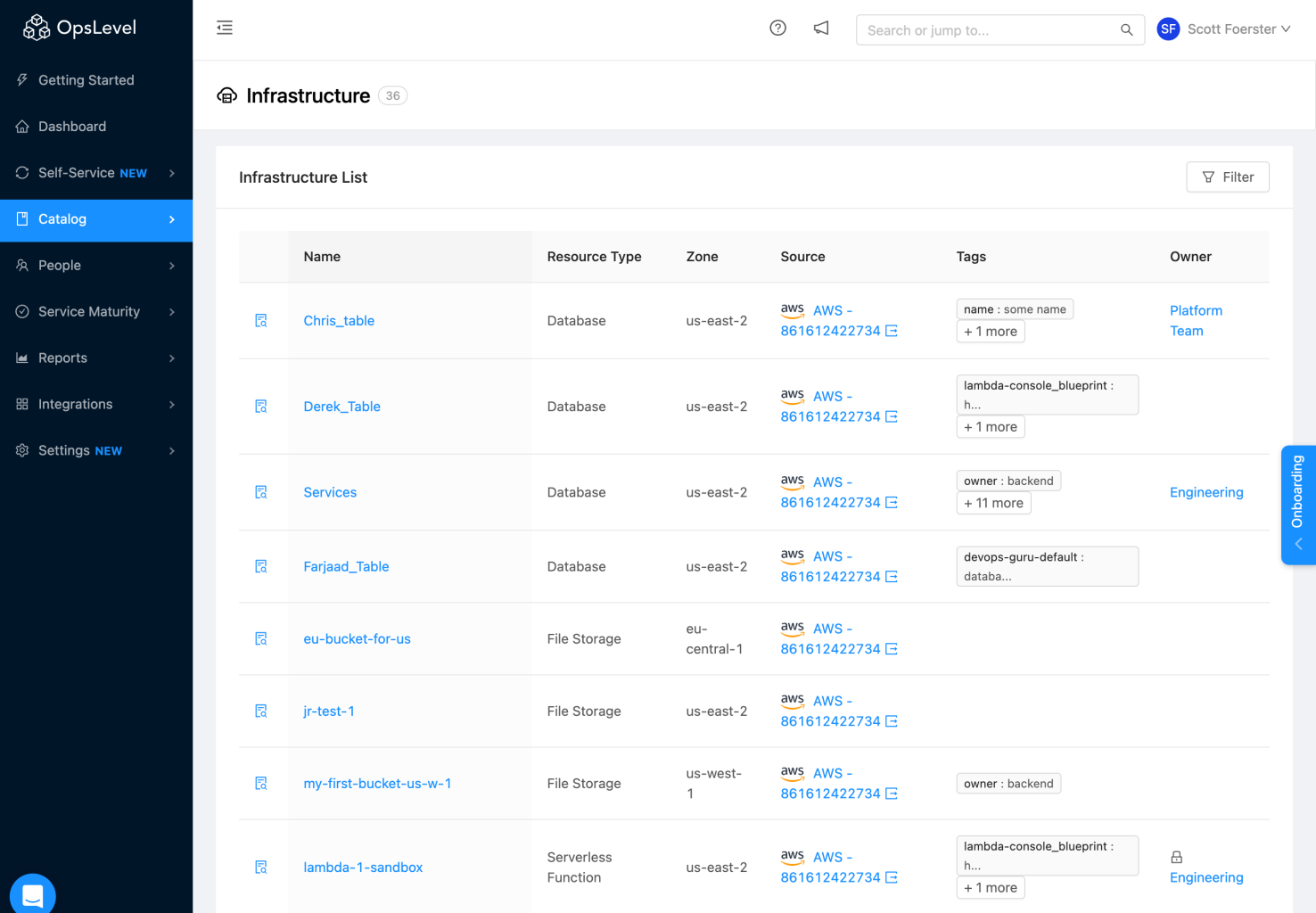
Infrastructure table
You can discover even more information about your Infrastructure Objects by clicking the View Details icon in the table. This will open a drawer component that will display when the object was last synced along with a host of attributes.
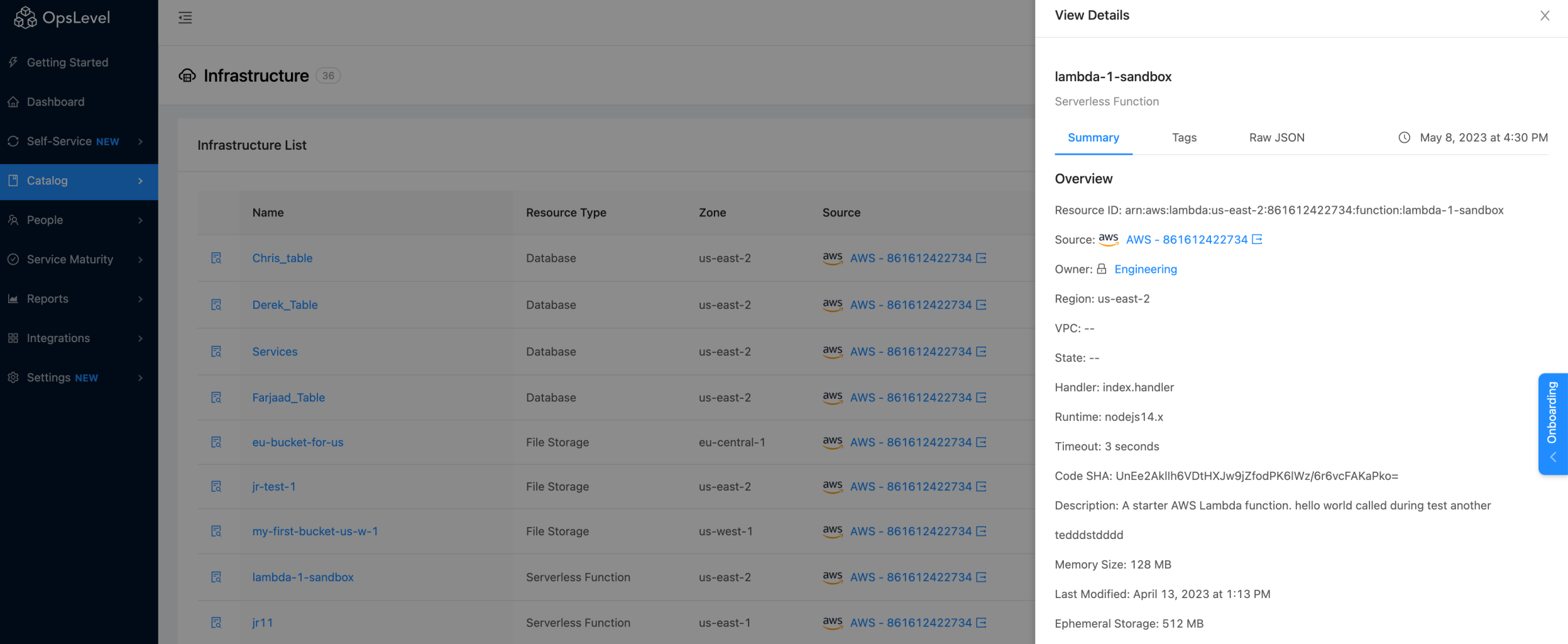
"View Details" drawer
For a full breakdown of what we've imported from your account, you can select the Raw JSON tab.
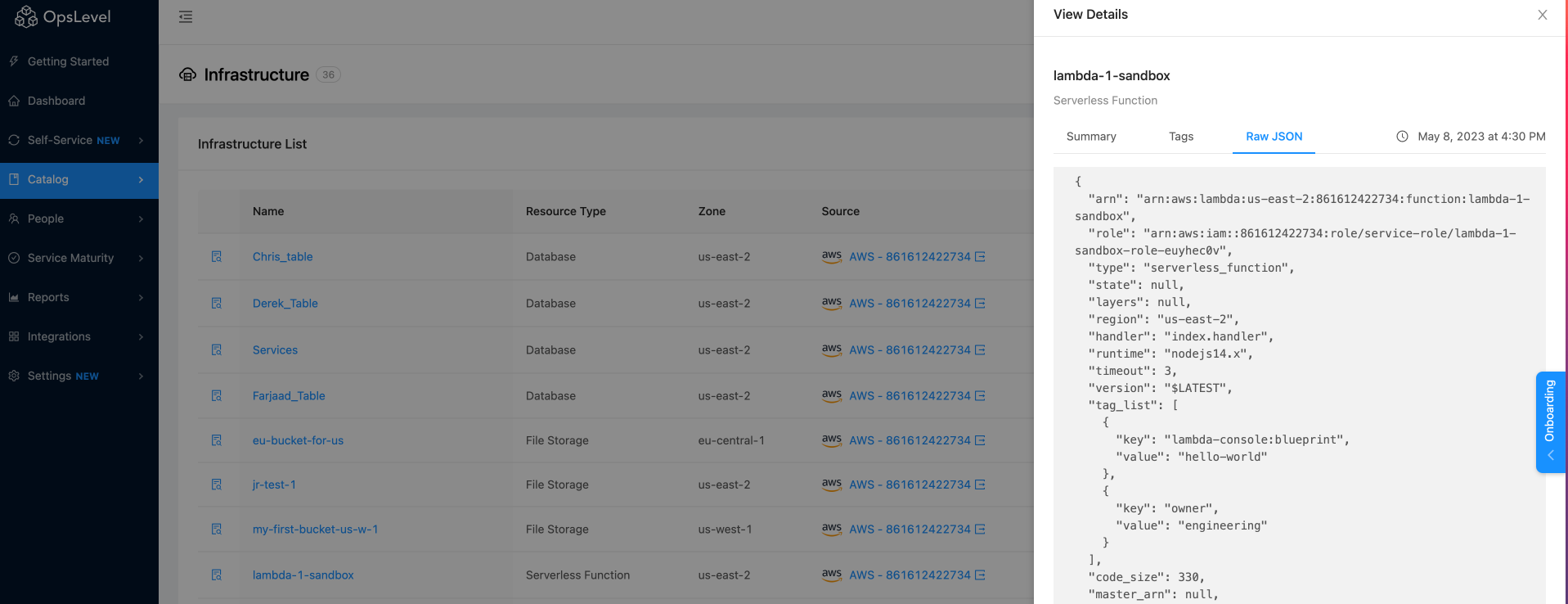
Raw JSON tab
Selecting the name of the object from the list will open a new details page for this object. The Infrastructure Details page contains all of the information displayed in the View Details drawer with the added bonus that you can also see which Services and other Infrastructure your infrastructure object relates to. See "Adding Relationships to Infrastructure Objects" further down in the docs.
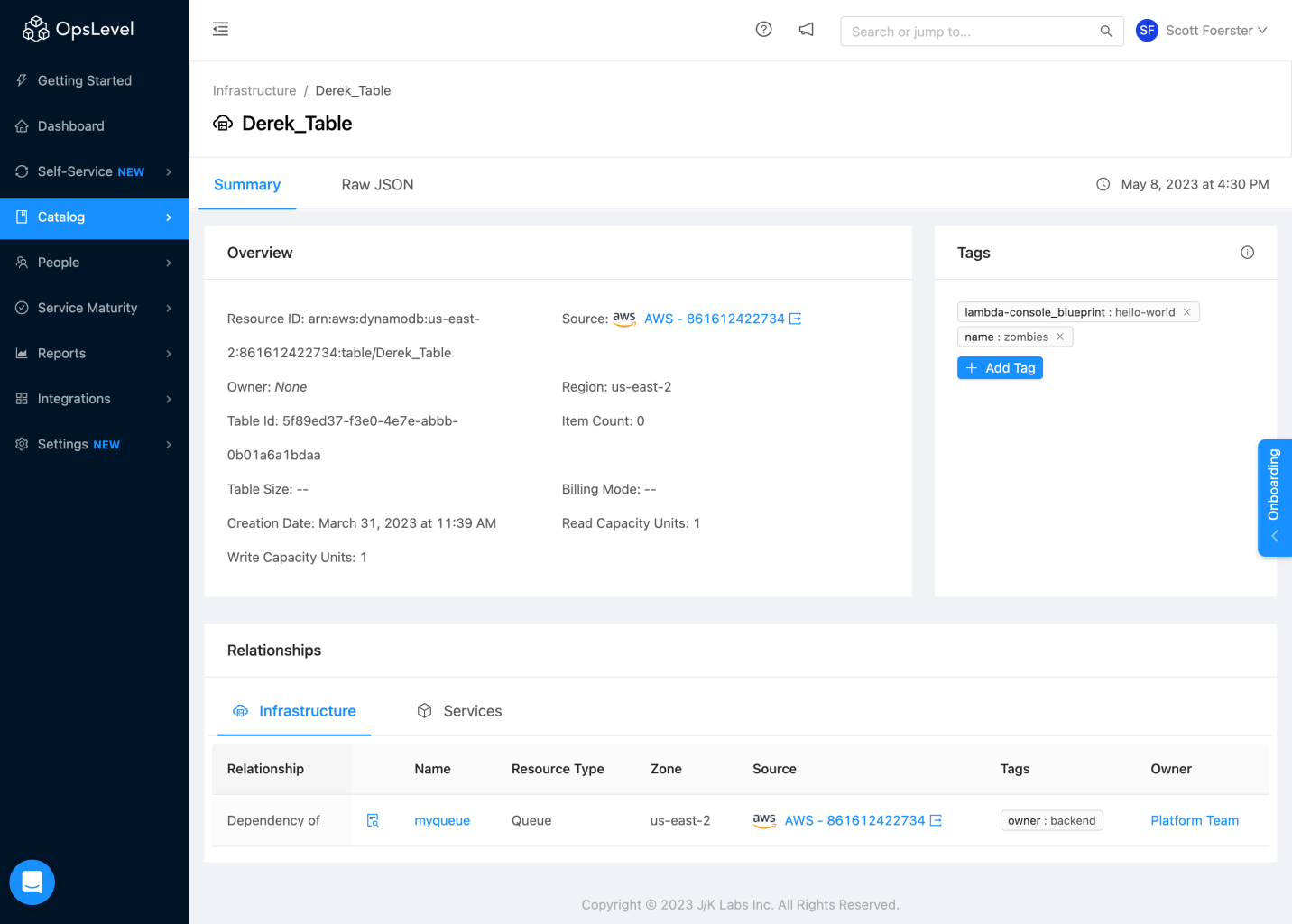
Infrastructure Details page
Filtering on Infrastructure objects
Similar to Services, you can apply filters against your list of Infrastructure Objects. Click the Filter icon from the Infrastructure List page to open filters. Filters work the same for Infrastructure as they do elsewhere in OpsLevel with the added bonus that you can filter on the attributes of your infrastructure objects. As such, you can filter for queries like "which of my databases have multi-zone availability enabled?".
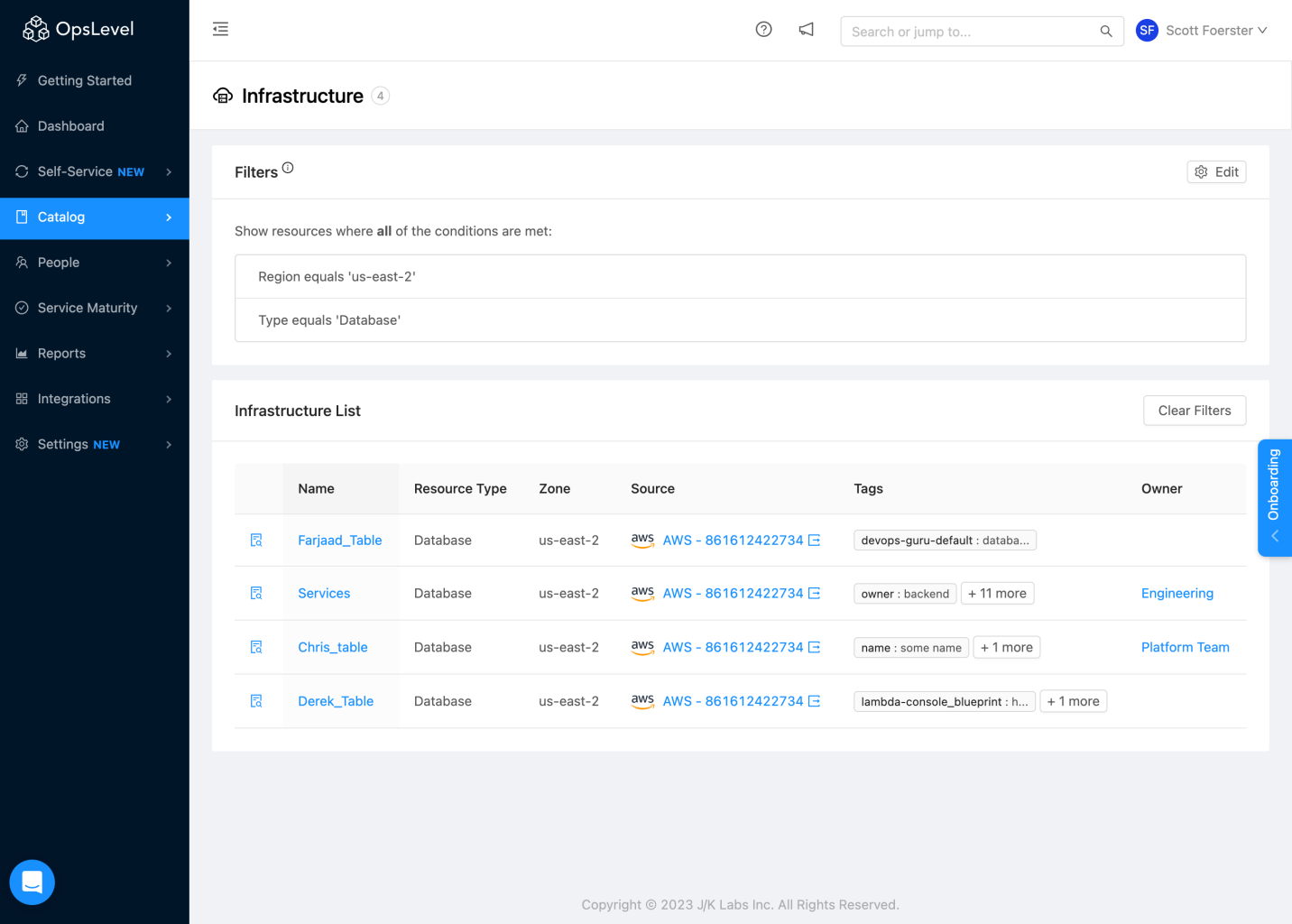
Filtering Infrastructure table
Adding relationships to Infrastructure objects
Utilizing GraphQL, you can add relationships to your Infrastructure Objects in three ways:
- "depends on"
- Infrastructure Objects can "depend on" Services or other Infrastructure Objects. A "depends on" relationship implies that the Infrastructure Object relies on the given Service or other Infrastructure Object to operate.
- "dependency of"
- Infrastructure Objects can be "dependent of" Services or other Infrastructure Objects. A "dependent of" relationship implies that the the Service(s) or other Infrastructure Object(s) related to your chosen Infrastructure Object rely on it to operate.
- "belongs to"
- Infrastructure Objects can belong to a System. Systems will show what Infrastructure Objects they contain.
Establishing relationships between Infrastructure objects and Services/Other Infrastructure objects
Using GraphQL, you can establish that a given Infrastructure Object can depend on or be the dependent of either another Infrastructure Object(s) and/or Service(s). See the following example in GraphQL:
mutation relationshipCreate($source: IdentifierInput!, $target: IdentifierInput!, $type: RelationshipTypeEnum!) {
relationshipCreate(relationshipDefinition: {
source: $source,
target: $target,
type: $type
}) {
relationship {
id
type
source {
... on Service {
id
name
__typename
}
... on System {
id
name
__typename
}
... on InfrastructureResource {
id
name
__typename
}
}
target {
... on Service {
id
name
__typename
}
... on System {
id
name
__typename
}
... on Domain {
id
name
__typename
}
... on InfrastructureResource {
id
name
__typename
}
}
}
errors {
message
path
}
}
}
Once you have set the relationship for your Infrastructure Object, you can see it in two places. The first is within the "Relationship" table in the Infrastructure Details page.
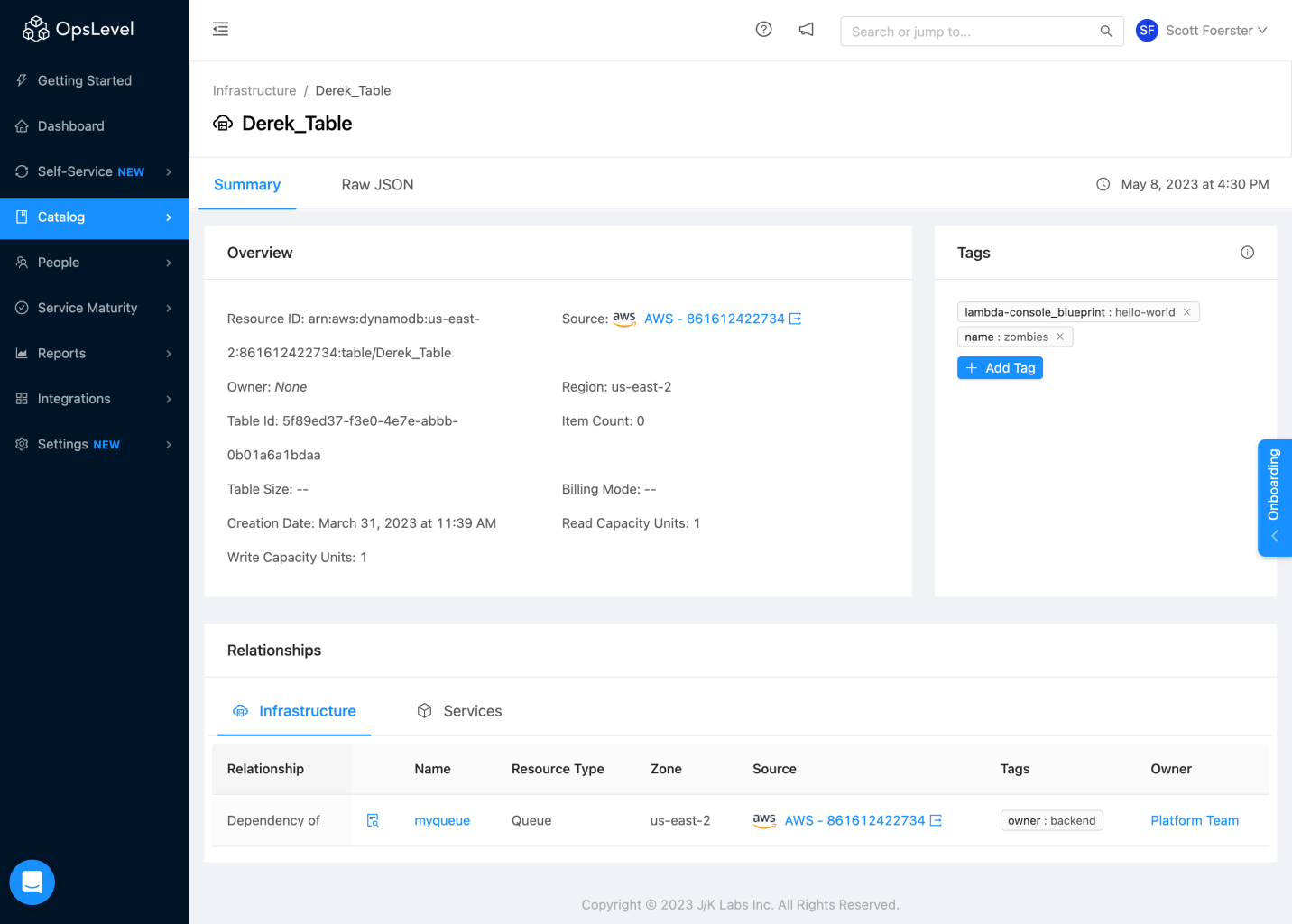
Relationship table in Infrastructure Details page
The second can be found by navigating to the Services show page and clicking on the Infrastructure tab under Dependencies
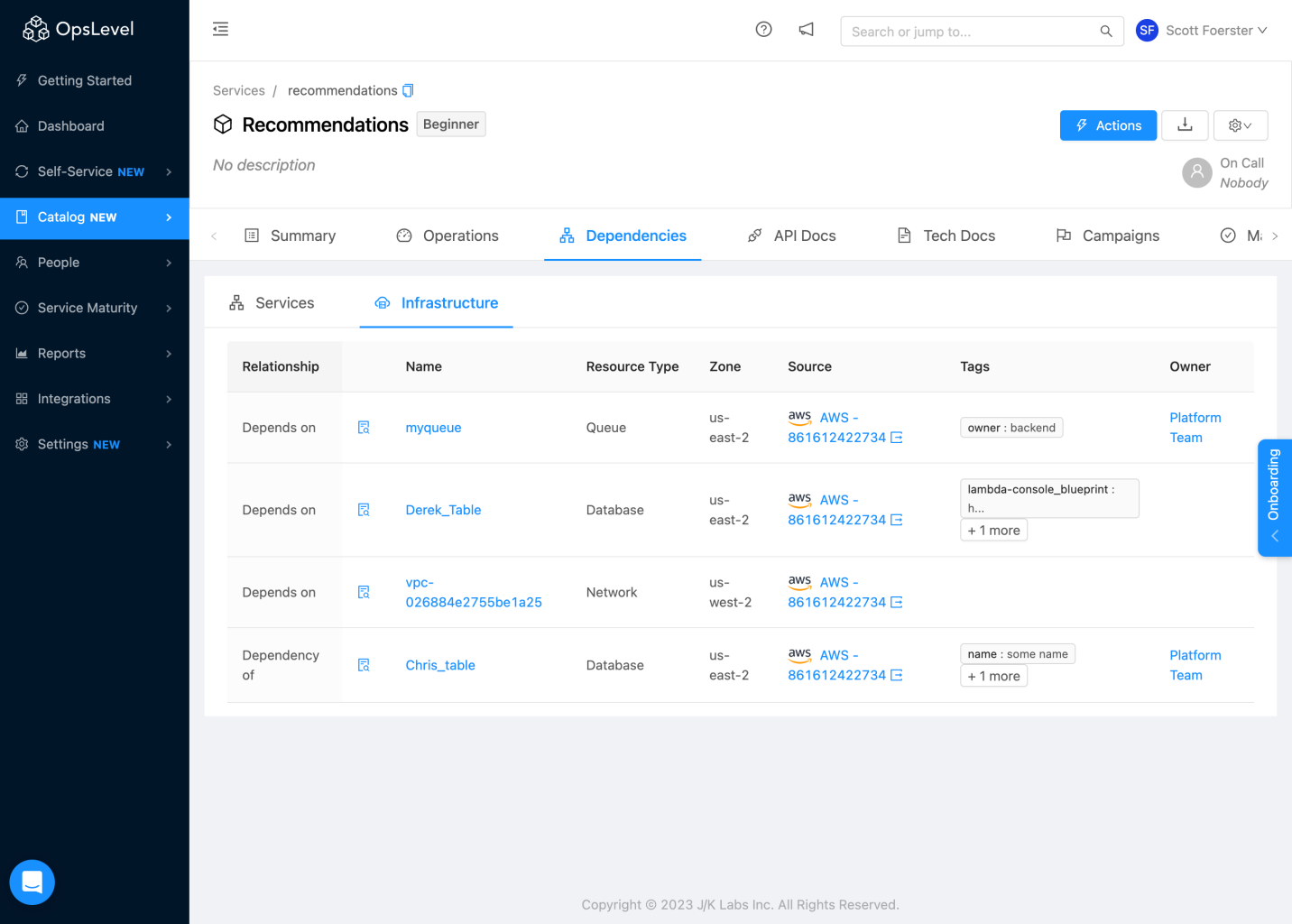
Infrastructure Dependency table
Establishing relationships between Infrastructure objects and Systems
Using GraphQL, you can establish which System a given Infrastructure Object belongs to. See the following example in GraphQL:
mutation relationshipCreate($source: IdentifierInput!, $target: IdentifierInput!, $type: RelationshipTypeEnum!) {
relationshipCreate(relationshipDefinition: {
source: $source,
target: $target,
type: $type
}) {
relationship {
id
type
source {
... on Service {
id
name
__typename
}
... on System {
id
name
__typename
}
... on InfrastructureResource {
id
name
__typename
}
}
target {
... on Service {
id
name
__typename
}
... on System {
id
name
__typename
}
... on Domain {
id
name
__typename
}
... on InfrastructureResource {
id
name
__typename
}
}
}
errors {
message
path
}
}
}
Once you have set the relationship between your Infrastructure Object and System, you will be able to see that your Infrastructure Object now belongs to the System by navigating to the Systems Display page and clicking on the Infrastructure tab under Relationships
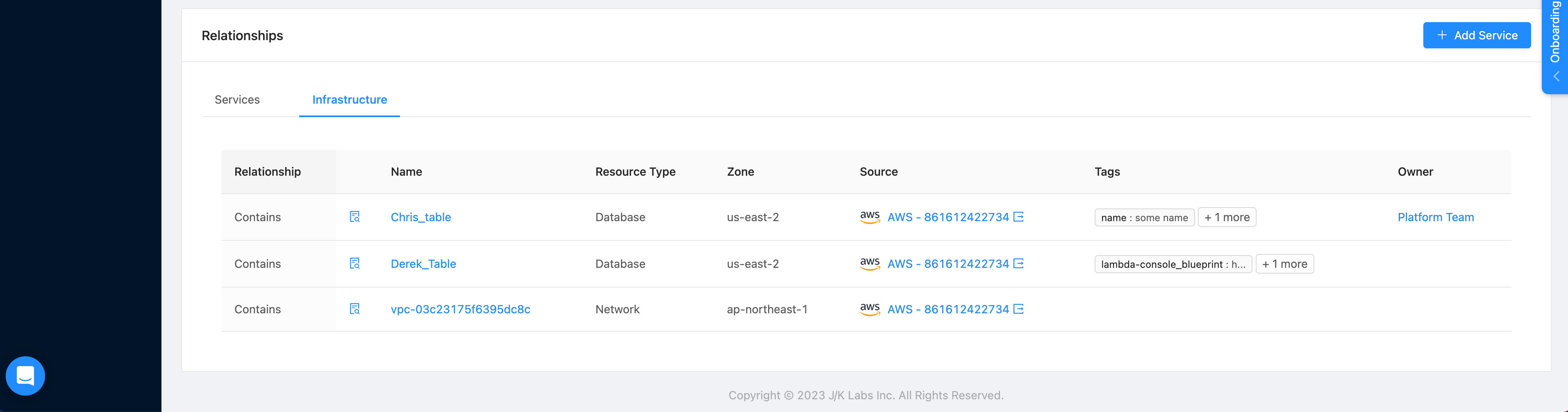
Systems Display Relationship table
Updated over 1 year ago