Advanced Usage and Reference
Create Filters and Checks based on custom properties.
Service Maturity
Custom Service Properties is available for use through our Service Maturity offering:
Filters
Custom Service Properties can be used within filters by first selecting Property
and then the desired Property Definition, followed by a jq expression to apply the correct filter.
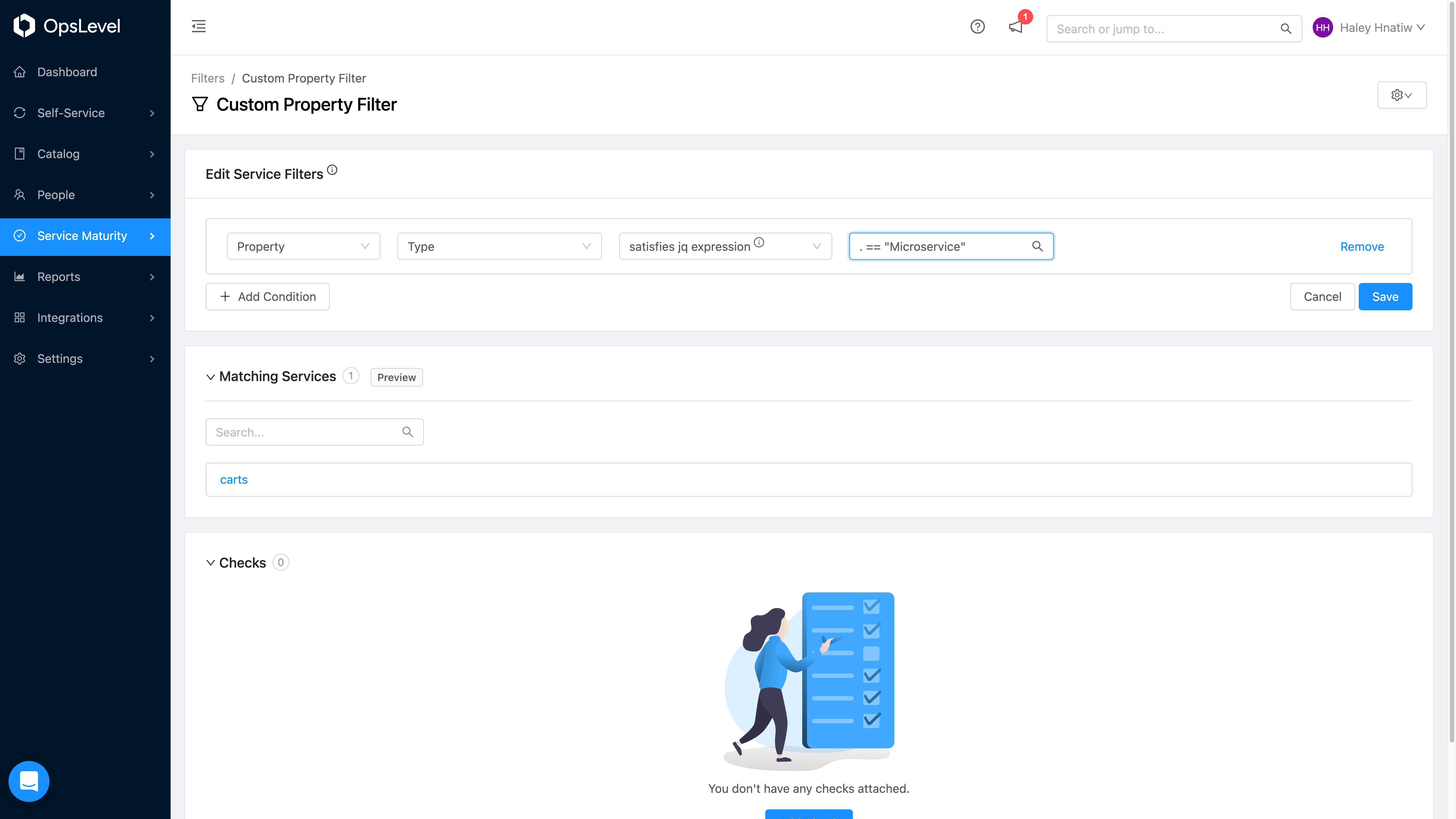
Custom service property filter creation page
Service Property Checks
Our existing Service Property Check has been extended to support Property Definition values. You can leverage the existing check, selecting a Property Definition name for the “Property” field under the Custom
group. By default the checks will ensure a value has been assigned to each service the check applies to. Optionally you can also apply a predicate in the “Advanced” section to write more custom validation.
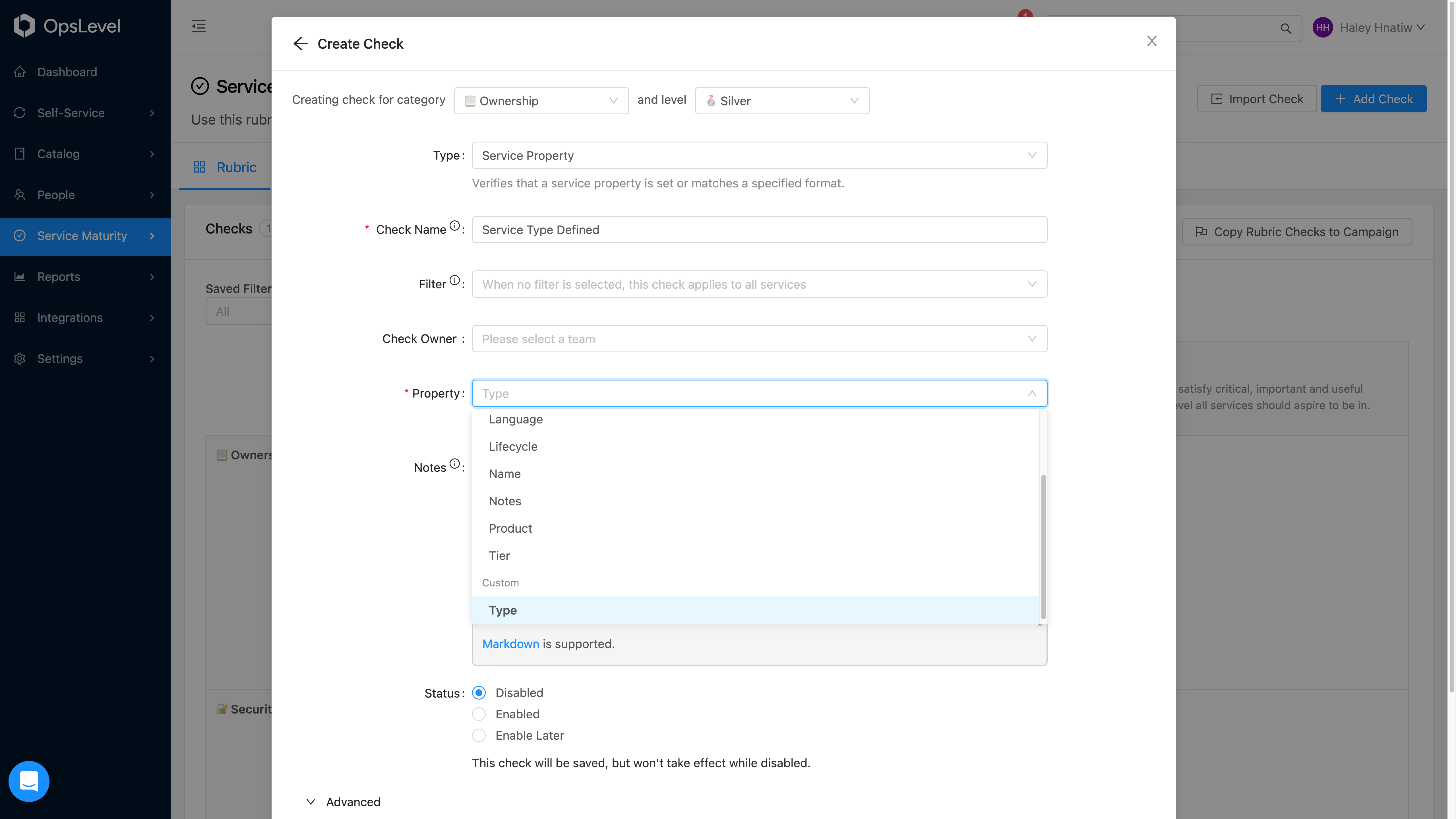
Configuring a Service Property Check on a custom property
API Reference
Below are a series of common API requests which can be used to manage Property Definitions or Service Property values
Property Definitions
Create
mutation {
propertyDefinitionCreate(input: {
name: "Latest Release",
schema: "{ \"type\": \"string\", \"format\": \"date-time\" }"
}){
definition {
id
schema
name
aliases
}
errors{
path
message
}
}
}
Update
mutation {
propertyDefinitionUpdate(
properyDefinition: { alias: "latest_release" },
input: {
schema: "{ \"type\": \"string\", \"format\": \"date-time\" }"
}
){
definition {
id
schema
name
aliases
}
errors{
path
message
}
}
}
Delete
mutation {
propertyDefinitionDelete(resource: {alias: "latest_release"}){
deletedId
errors {
path
message
}
}
}
List Properties
query {
account {
propertyDefinitions {
nodes {
id
name
aliases
schema
}
}
}
}
Service Properties
Property Assign
mutation {
propertyAssign(input: {
owner: { alias: "music_catalogue"},
definition: { alias: "latest_release"},
value: "\"2023-10-12T20:20:39+00:00\""
}){
property {
value
owner {
__typename
...on Service {
name
}
}
}
errors {
path
message
}
}
}
Property Un-Assign
mutation {
propertyUnassign(
owner: { alias: "music_catalogue"},
definition: { alias: "latest_release"}
){
definition {
name
}
owner {
__typename
...on Service {
name
}
}
errors {
path
message
}
}
}
List Property Values on a service
query list {
account {
service(alias: "music_catalogue") {
properties {
nodes {
value
definition {
name
schema
aliases
}
}
}
}
}
}
Get Property Value
query get {
account {
property(
owner: { alias: "music_catalogue"},
definition: { alias: "latest_release"}
) {
value
}
}
}
Filtering Reference
For details, take a look at the JQ language documentation.
Property Exists / Does Not Exist
// exists
.
// does not exist
. | not
Boolean
. == true
String
// matches "some value"
. == "some value"
// matches all other values that are not "some value" and the property is set
. != "some value"
Number
. > 4
Object
.key == "value"
Array
// map through the array to check for values
.[] | . == true
// pick a particular index
.[0] | . == true
// Array contains value
any(. == true)
// Array contains object with property value
any(.severity == "high")
Advanced Examples
JQ is very expressive and allows very complex queries. Keep in mind that we’re always interpreting the result of the expression as either false or true.
// Array contains more than 4 items with high severity
reduce .[] as $item (0; if $item.severity == "high" then . + 1 else . end) > 4
Sample Data
Not sure how to get started with using service properties? We have you covered! Below is a list of different objects we thought you’d like to track
Vulnerabilities
Track a list of vulnerabilities that are linked to your service
{
"title": "Vulnerabilities",
"description": "A list of vulnerable packages",
"type": "array",
"items": {
"type": "object",
"properties": {
"name": { "type": "string" },
"severity": {
"type": "string",
"enum": ["low", "medium", "high", "critical"]
},
"introduced_through": { "type": "string" },
"fixed_in": { "type": "string" }
},
"required": ["name", "severity"]
},
"minItems": 0,
"uniqueItems": true
}
Packages
{
"title": "Packages",
"description": "A list of packages.",
"type": "array",
"items": {
"type": "object",
"properties": {
"name": { "type": "string" },
"version": { "type": "string" },
"lock_file": { "type": "string" },
"manager": { "type": "string" }
},
"required": ["name", "version"]
},
"minItems": 0,
"uniqueItems": true
}
Environments
{
"title": "Environments",
"description": "The different environments a service can be deployed to.",
"type": "string",
"enum": ["prod", "dev"]
}
Publicly Routable
{
"title": "Publicly Routable",
"description": "Can the service be accessed through the public internet?",
"type": "boolean"
}
Updated 5 months ago