Configuring Actions Manual Inputs
Manual inputs allow users to add contextual information to an action as it is being invoked. They are defined using YAML markup. The screenshot below shows an example of manual inputs configured for an action that allows a developer to provision an S3 bucket:
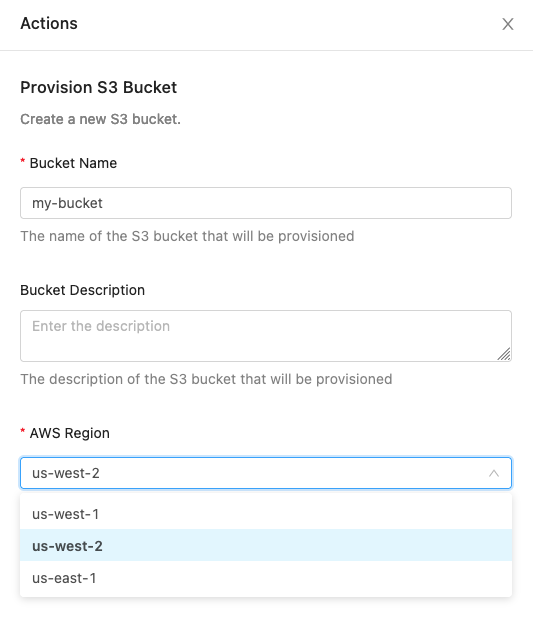
The schema supports two root level keys:
Description | |
---|---|
version | The schema version (current version: 1). |
inputs | A list of input objects. See the Input Object Schema for more information. |
Example configuration (this configuration is the yaml that drives the example screenshot above):
---
version: 1
inputs:
- identifier: bucketName
required: true
type: text_input
displayName: Bucket Name
description: The name of the S3 bucket that will be provisioned
maxLength: 20
defaultValue: my-bucket
pattern: "^[\\w-]+$" # Note: the escaped backslash.
- identifier: bucketDescription
required: false
type: text_area
displayName: Bucket Description
description: The description of the S3 bucket that will be provisioned
placeholder: Enter the description
- identifier: region
required: true
type: dropdown
displayName: AWS Region
description: The AWS Region that the bucket will be provisioned in
defaultValue: us-west-2
values:
- us-west-1
- us-west-2
- us-east-1
Input object schema
Inputs support the following properties:
Required | Description | |
---|---|---|
identifier | true | Define how to reference the input’s value from the action’s context. |
label | true | The input title displayed to the invoking user. |
type | true | Supported types: text_input , text_area , and dropdown . |
defaultValue | false | The initial value for this input. |
description | false | Additional information displayed to the invoking user. This field supports Markdown. |
maxLength | false | The invoking user will receive an error if they invoke the action with a value longer than maxLength. |
pattern | false | The invoking user will receive an error if they attempt to submit an input which does not match this regular expression. Note: Backslashes must be escaped. \\w must be written as \\\\w . |
placeholder | false | The input placeholder displayed to the invoking user. Note: Only available on inputs of type text_input and .text_area . |
required | false | The invoking user will receive an error if they invoke the action without providing a value to this input. |
sensitive | false | Indicates the field contains a sensitive value (e.g. a password). The value will be obfuscated when executing the action and when viewing the execution history. Note: Only available on inputs of type text_input . |
values | false | The select options available to the invoking user. Note: Only available on inputs of type dropdown . |
Values Schema
The values
array allows for two methods of configuration:
-
Value only:
values:
- value1
- value2
-
Value and Display:
values:
- value: value1
display: display 1 - value: value2
display: display 2
- value: value1
Dropdown configuration examples:
---
version: 1
inputs:
- identifier: value_only_example
displayName: Value Only Example
description: This input shows a dropdown using value-only configurations
type: dropdown
values:
- us-west-1
- us-west-2
- us-east-1
defaultValue: us-west-2
required: true
- identifier: value_and_display_example
displayName: Value And Display Example
description: This input shows a dropdown using value and display configurations
type: dropdown
values:
- value: us-west-1
display: US West 1
- value: us-west-2
display: US West 2
- value: us-east-1
display: US East
defaultValue: us-west-2
required: true
- identifier: mixed_example
displayName: Mixed Example
description: This input shows a dropdown using a mix of value-only and value and display configurations
type: dropdown
values:
- value: us-west-1
display: US West Primary
- us-west-2
- value: us-east-1
display: US East
defaultValue: us-west-2
required: true
Updated about 1 year ago